Search results
Disabling Redis Auto-configuration in Spring Boot applications
1. OVERVIEW
I was recently working on a Spring Boot web application where it was decided to reduce external services dependencies to speedup development, caching using Redis in this specific case.
The interaction between the web application and a Redis server is done using Spring’s RedisTemplate, an implemention of the Template design pattern, used similarly to RestTemplate, JdbcTemplate, JmsTemplate, etc..
A RedisTemplate and related beans are auto-configured by Spring Boot when including spring-boot-starter-data-redis as a dependency for the application. This posts covers some tips to prevent such connection from being auto-configured.
2. EXCLUDING RedisAutoConfiguration
In general Spring Boot beans auto-configuration could be disabled or prevented when excluding them through properties or via annotation:
application.yml
...
spring:
redis:
host: localhost
port: 6379
---
spring:
profiles: no-redis
autoconfigure:
exclude:
- org.springframework.boot.autoconfigure.data.redis.RedisAutoConfiguration
...
Redis connection-related beans won’t get auto-configured when using the Spring profile no-redis
.
exclude
attribute of the @SpringBootApplication annotation, something like:
@SpringBootApplication( exclude = { RedisAutoConfiguration.class } )
But running the application using spring.profiles.active=no-redis
fails to initialize the Spring context due to this exception:
...
2017-10-12 22:30:39 WARN AnnotationConfigEmbeddedWebApplicationContext:551 - Exception encountered during context initialization - cancelling refresh attempt: org.springframework.beans.factory.BeanCreationException: Error creating bean with name 'redisReferenceResolver': Cannot resolve reference to bean 'redisTemplate' while setting constructor argument; nested exception is org.springframework.beans.factory.NoSuchBeanDefinitionException: No bean named 'redisTemplate' available
...
Even though it gets logged as a WARN
, the application doesn’t start.
3. SOLVING THE ISSUE
After some troubleshooting, it is not only required to exclude RedisAutoConfiguration but also RedisRepositoriesAutoConfiguration, which could be accomplished in two ways:
3.1. PREVENTING RedisRepositoriesAutoConfiguration AUTO-CONFIGURATION USING exclude
Via properties file:
...
spring:
autoconfigure:
exclude:
- org.springframework.boot.autoconfigure.data.redis.RedisAutoConfiguration
+ - org.springframework.boot.autoconfigure.data.redis.RedisRepositoriesAutoConfiguration
..
or via @SpringBootApplication annotation:
...
@SpringBootApplication(
exclude = { RedisAutoConfiguration.class }
- exclude = { RedisAutoConfiguration.class }
+ exclude = { RedisAutoConfiguration.class, RedisRepositoriesAutoConfiguration.class }
)
...
3.2. PREVENTING RedisRepositoriesAutoConfiguration AUTO-CONFIGURATION USING REDIS-SPECIFIC PROPERTY
...
+spring.data.redis.repositories.enabled: false
...
Thanks for reading and as always, feedback is very much appreciated. If you found this post helpful and would like to receive updates when content like this gets published, sign up to the newsletter.
4. SOURCE CODE
Accompanying source code for this blog post can be found at:
5. REFERENCES
NEED HELP?
I provide Consulting Services.ABOUT THE AUTHOR
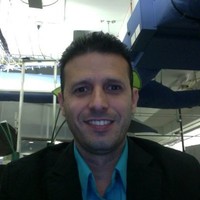