Search results
Pushing Spring Boot 2 Docker images to Microsoft ACR
1. OVERVIEW
Google’s jib-maven-plugin, Spotify’s docker-maven-plugin, and spring-boot-maven-plugin since Spring Boot 2.3 help you to build Docker images for your Spring Boot applications.
You might also want to store these Docker images in a private Docker registry.
Microsoft’s Azure Container Registry (ACR) is a cheap option to store both, public and private Docker images.
It would even makes more sense to use ACR if your organization is already invested in other Azure services.
Spring Boot application in a Docker image stored in a private Azure Container Registry
This tutorial covers setting up the Azure infrastructure and Maven configuration to push a Docker image to a private ACR repository.
2. AZURE-CLI
az --version
azure-cli 2.50.0 *
...
This blog post uses azure-cli
version 2.50.0
to provision the resources to host your Docker images in a private ACR repository.
It doesn’t cover how to install Azure CLI. Please refer to https://learn.microsoft.com/en-us/cli/azure/install-azure-cli to install it for your relevant OS.
3. SETUP THE AZURE INFRASTRUCTURE
We’ll run a number of azure-cli
commands to provisions all the resources to store Docker images.
3.1. Login to Azure
az login
A web browser has been opened at https://login.microsoftonline.com/organizations/oauth2/v2.0/authorize. Please continue the login in the web browser. If no web browser is available or if the web browser fails to open, use device code flow with `az login --use-device-code`.
A browser tab opens with Microsoft Azure Login page. Please login using the Web UI. You can close the tab afterwards.
Once you login, there should be a JSON response in the terminal window like:
[
{
"cloudName": "AzureCloud",
"homeTenantId": "xxxxxxxxxxxxxxx",
"id": "<Your Azure subscription id>"
"name": "<Your Azure subscription name>",
"tenantId": "xxxxxxxxxxxxxxx",
"user": {
...
}
...
}
]
3.2. Set the Azure subscription
az account set -s <Your Azure subscription id>
id
value from the az login command JSON response.
3.3. Create a resource group
If you don’t have one yet, you’ll need to create an Azure resource group to include different resources.
az group create --name=<Your resource group name> --location=eastus
I used eastus
for the location, but you can find other Azure locations using azure-cli
:
az account list-locations
and use the name
value from the desired array element as the location for your Azure resource group.
If you would like to reuse a resource group and its location, your could retrieve these values using azure-cli
:
az group list
3.4. Create a private Docker registry
az acr create --resource-group <Your resource group name> --location <location> --name <Your registry name> --sku Basic
Replace <Your resource group name> and <location> with the values you used to create the resource group, or with the values retrieved from an existing resource group.
Replace also <Your registry name> with the name you intend to give to your Docker registry.
After creating the Docker registry, the terminal might display a message along with a JSON-formatted response similar to:
Resource provider 'Microsoft.ContainerRegistry' used by this operation is not registered. We are registering for you.
Registration succeeded.
{
"adminUserEnabled": false,
"anonymousPullEnabled": false,
"id": "/subscriptions/<Your Azure subscription id>/resourceGroups/<Your resource group name>/providers/Microsoft.ContainerRegistry/registries/<Your registry name>",
"location": "eastus",
"loginServer": "<Your registry name>.azurecr.io",
"name": "<Your registry name>",
...
}
Now that the Azure infrastructure is setup, let’s configure Maven to push a Docker image with a Spring Boot application to this Azure Container Registry.
4. MAVEN CONFIGURATION
Let’s add the jib-maven-plugin configuration to pom.xml
.
pom.xml
:
...
<plugin>
<groupId>com.google.cloud.tools</groupId>
<artifactId>jib-maven-plugin</artifactId>
<version>3.3.2</version>
<configuration>
<container>
<mainClass>com.asimiotech.demo.Application</mainClass>
</container>
<from>
<image>mcr.microsoft.com/java/jdk:11-zulu-alpine</image>
</from>
<to>
<image><Your registry name>.azurecr.io/${project.artifactId}:${project.version}</image>
</to>
</configuration>
</plugin>
...
If the mainClass
is not inferred, you would need to add it to the jib-maven-plugin
configuration.
5. BUILD AND PUSH THE SPRING BOOT DOCKER IMAGE
Before pushing a Docker image to the registry, we need to login to the Azure Container Registry that we just created.
Let’s first generate an access token using azure-cli
:
TOKEN=$(az acr login --name <Your registry name> --expose-token --output tsv --query accessToken)
Let’s now login to the Container Registry using this access token:
docker login <Your registry name>.azurecr.io -u 00000000-0000-0000-0000-000000000000 --password-stdin <<< $TOKEN
You are ready to build and push the Spring Boot application Docker image:
mvn -f pom-azure-docker-acr.xml --debug jib:build
...
[INFO] Built and pushed image as asimiodockerregistry.azurecr.io/springboot2-logback-json:0-SNAPSHOT
[INFO] Executing tasks:
[INFO] [============================ ] 91.7% complete
[INFO] > launching layer pushers
[INFO]
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
Login to the Azure Console UI and browsing through Container Registries you’ll find the new Docker image.
Pushed a Docker image to an Azure Container Registry
6. PULL THE SPRING BOOT DOCKER IMAGE
You can now test pulling this Docker image.
docker pull <Your registry name>.azurecr.io/springboot2-logback-json:0-SNAPSHOT
If you get a response similar to:
Error response from daemon: Head "https://<Your registry name>.azurecr.io/v2/springboot2-logback-json/manifests/0-SNAPSHOT": unauthorized: authentication required
you would need to generate a new access token and login to the Azure Container Registry again, and retry pulling the image.
Once the docker pull
command completes, your terminal output should look like:
...
Digest: sha256:c9eeabaa3ad74c48c46e575c315a127845e31527f0d1ae920858411ca2f5cbd7
Status: Downloaded newer image for <Your registry name>.azurecr.io/springboot2-logback-json:0-SNAPSHOT
<Your registry name>.azurecr.io/springboot2-logback-json:0-SNAPSHOT
...
7. CONCLUSION
Hosting your Docker images in Azure Container Registry repositories is a very good option if your organization is using, or planning to use other Azure services.
This blog post helped you to setup the Azure infrastructure to host your Docker images in a private Container Registry.
It also helped you with the Maven configuration to push a Spring Boot Docker image to ACR.
Thanks for reading and as always, feedback is very much appreciated. If you found this post helpful and would like to receive updates when content like this gets published, sign up to the newsletter.
8. SOURCE CODE
Accompanying source code for this blog post can be found at:
9. REFERENCES
NEED HELP?
I provide Consulting Services.ABOUT THE AUTHOR
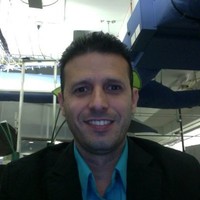