Search results
Uploading JaCoCo Code Coverage Reports to SonarQube
1. OVERVIEW
SonarQube is a widely adopted tool that collects, analyses, aggregates and reports the source code quality of your applications.
It helps teams to measure the quality of the source code as your applications mature.
SonarQube integrates with popular CI/CD tools so that you can get source code quality reports every time a new application build is triggered; helping teams to fix errors, reduce technical debt, maintain a clean source base, etc.
A previous blog post covered how to generate code coverage reports using Maven and JaCoCo.
This blog post covers how to generate JaCoCo code coverage reports and upload them to SonarQube.
SonarQube Overall Code Quality - Static Code Analysis
2. MAVEN CONFIGURATION
The accompanying source code has one unit test and two integration tests.
Unit Tests | Integration Tests |
DemoControllerTest.java | ApplicationTests.java |
DemoControllerIT.java |
Let’s configure the maven-surefire-plugin and jacoco-maven-plugin Maven plugins in a custom Maven POM file.
pom-no-tests-splitting.xml
POM file because this same source code is discussed in another blog post and these are breaking changes with what’s being discussed there.
pom-no-tests-splitting.xml
:
...
<properties>
<java.version>11</java.version>
</properties>
...
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<configuration>
<skipTests>false</skipTests>
<includes>
<include>**/*Test.java</include>
<include>**/*Tests.java</include>
<include>**/*IT.java</include>
</includes>
</configuration>
</plugin>
<plugin>
<groupId>org.jacoco</groupId>
<artifactId>jacoco-maven-plugin</artifactId>
<version>0.8.10</version>
<executions>
<execution>
<id>coverage-initialize</id>
<goals>
<goal>prepare-agent</goal>
</goals>
</execution>
<execution>
<id>jacoco-report</id>
<phase>package</phase>
<goals>
<goal>report</goal>
</goals>
</execution>
</executions>
</plugin>
...
If your test classes names end with suffixes Test, or Tests, you can leave maven-surefire-plugin’s includes
section out.
I added the includes
section because the source code includes test classes with the IT suffix.
With this maven-surefire-plugin’s configuration, the package
phase will also execute the test
phase.
The jacoco-maven-plugin executes the report
goal during the package
phase.
It generates target/jacoco.exec
binary report file. You can find reports in other formats under target/site/jacoco/
report
goal during the post-integration-test
phase so that it generates reports for both, unit and integration tests.
3. BUILD THE ARTIFACT AND JACOCO REPORTS
mvn -f pom-no-tests-splitting.xml clean package
...
[INFO] --- jacoco-maven-plugin:0.8.10:report (jacoco-report) @ code-coverage-maven-jacoco ---
[INFO] Loading execution data file /Users/ootero/Projects/bitbucket.org/code-coverage-maven-jacoco/target/jacoco.exec
[INFO] Analyzed bundle 'code-coverage-maven-jacoco' with 3 classes
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
...
The Maven command uses a custom Maven POM file pom-no-tests-splitting.xml.
It compiles, runs the unit and integration tests, generates the binary artifact and the JaCoCo reports:
ls target/ target/site/jacoco/
target/:
...
jacoco.exec
...
target/site/jacoco/:
com.asimio.demo
com.asimio.demo.rest
com.asimio.demo.service
index.html
jacoco-resources
jacoco-sessions.html
jacoco.csv
jacoco.xml
4. STARTING A SONARQUBE INSTANCE
You first need a running SonarQube instance.
If you don’t have one you could start a Docker container for testing purposes using the sonarqube:lts-community
image:
docker run -d --name sonarqube -p 9000:9000 sonarqube:lts-community
...
c0de85910c9ea358830e5327c2fb06e5d21a252185afef070bc0b5388d2251c1
docker logs c0de85
...
2023.07.05 17:48:25 INFO ce[][o.s.c.c.ComputeEngineContainerImpl] Running Community edition
2023.07.05 17:48:25 INFO ce[][o.s.ce.app.CeServer] Compute Engine is started
2023.07.05 17:48:26 INFO app[][o.s.a.SchedulerImpl] Process[ce] is up
2023.07.05 17:48:26 INFO app[][o.s.a.SchedulerImpl] SonarQube is operational
You can access SonarQube’s UI at http://localhost:9000/
.
Log in using:
Username | Password |
admin | admin |
and the first screen that comes up after loging in prompts you to change the password. I changed it to sonar
.
5. UPLOAD JACOCO REPORTS TO SONARQUBE
Now that you have a running SonarQube instance, the next step in your CI/CD build pipeline would be to upload the JaCoCo reports.
mvn sonar:sonar -Dsonar.projectKey=code-coverage-maven-jacoco -Dsonar.coverage.jacoco.xmlReportPaths=target/site/jacoco/jacoco.xml -Dsonar.host.url=http://localhost:9000 -Dsonar.login=admin -Dsonar.password=sonar
...
[INFO] Sensor JaCoCo XML Report Importer [jacoco]
[INFO] Importing 1 report(s). Turn your logs in debug mode in order to see the exhaustive list.
[INFO] Sensor JaCoCo XML Report Importer [jacoco] (done) | time=74ms
...
[INFO] Analysis report generated in 78ms, dir size=118 KB
[INFO] Analysis report compressed in 49ms, zip size=28 KB
[INFO] Analysis report uploaded in 203ms
[INFO] ANALYSIS SUCCESSFUL, you can browse http://localhost:9000/dashboard?id=code-coverage-maven-jacoco
[INFO] Note that you will be able to access the updated dashboard once the server has processed the submitted analysis report
[INFO] More about the report processing at http://localhost:9000/api/ce/task?id=AX7ggEQ4mh3uJDOtN12t
[INFO] Analysis total time: 11.205 s
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
...
If you get this error:
[ERROR] Failed to execute goal org.sonarsource.scanner.maven:sonar-maven-plugin:3.7.0.1746:sonar (default-cli) on project code-coverage-maven-jacoco: Execution default-cli of goal org.sonarsource.scanner.maven:sonar-maven-plugin:3.7.0.1746:sonar failed: An API incompatibility was encountered while executing org.sonarsource.scanner.maven:sonar-maven-plugin:3.7.0.1746:sonar: java.lang.UnsupportedClassVersionError: org/sonar/batch/bootstrapper/EnvironmentInformation has been compiled by a more recent version of the Java Runtime (class file version 55.0), this version of the Java Runtime only recognizes class file versions up to 52.0
Try to upgrade your Java version to 11 or higher in your Maven POM file.
Some of the command parameters are:
sonar.projectKey | The project’s unique key. The artifactId for Maven’s projects. |
sonar.coverage.jacoco.xmlReportPaths. | The file path to JaCoCo reports in XML format. |
sonar.host.url | The SonarQube server’s URL. |
sonar.login | The SonarQube server’s username. |
sonar.password | The SonarQube server’s password. |
You can find more options at https://docs.sonarqube.org/latest/analysis/analysis-parameters/.
The SonarQube analysis UI for this Java application is available at http://localhost:9000/dashboard?id=code-coverage-maven-jacoco
.
SonarQube Issues
And the code coverage page:
SonarQube Coverage
6. CONCLUSION
SonarQube is a valuable and popular tool that helps engineering teams to keep a clean base code as the applications evolve.
You can upload JaCoCo code coverage reports to SonarQube as part of your CI/CD pipeline when building your Java applications.
Thanks for reading and as always, feedback is very much appreciated. If you found this post helpful and would like to receive updates when content like this gets published, sign up to the newsletter.
7. SOURCE CODE
Accompanying source code for this blog post can be found at:
NEED HELP?
I provide Consulting Services.ABOUT THE AUTHOR
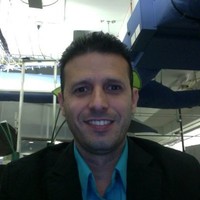